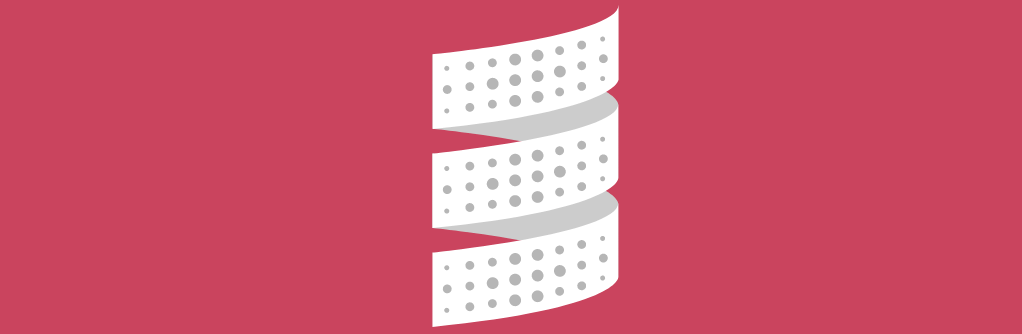
Pair
A pair include 2 elements that is written (x, y) in Scala
Pairs can also be used as patterns: val (label, value) = pair
Tuple
A tuple includes more than 2 elements
The fields of a tuple can be accessed with names _1, _2, …
Example
Write a function merge sort using pair and pair pattern
def msort(xs: List[Int]): List[Int] = { val n = xs.length/2 if (n == 0) xs else { val (first, second) = xs splitAt(n) merge(msort(first), msort(second)) } } def merge(xs: List[Int], ys: List[Int]): List[Int] = { (xs, ys) match { case (Nil, ys) => ys case (x :: xs1, Nil) => xs case (x :: xs1, y :: ys1) => if(x < y) x :: merge(xs1, y :: ys1) else y :: merge(x :: xs1, ys1) } }