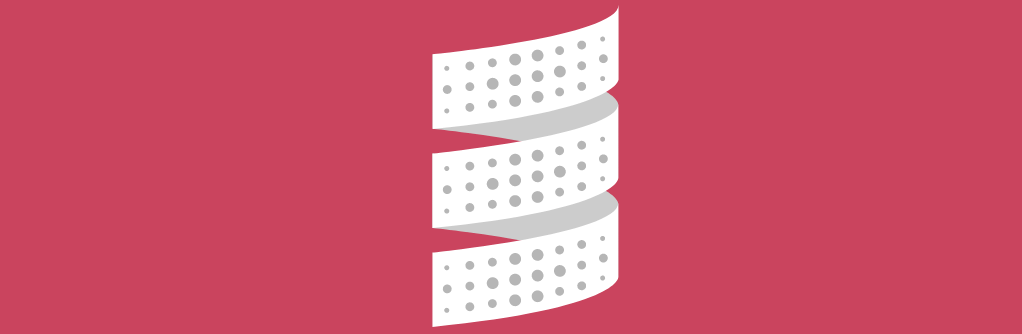
- The function type A => B is just an abbreviation for the class scala. Function1[A, B], which is defined as follows.
package scala; trait Function1[A, B] { def apply(x: A): B }
- An anonymous function such as
(x: Int) => x * x
is expanded to:
new Function1[Int, Int] { def apply(x: Int) = x * x }
- A function call, such as f(a), where f is a value of some class type, is expanded to f.apply(a)
val f = (x: Int) => x * x; f(7)
would be
val f = new Function1[Int, Int] { def apply(x: Int) = x * x } f.apply(7)
- A method such as
def f(x: Int): Boolean = ...
is not itself a function value.
But if f is used in a place where a Function type is expected, it is converted automatically to the function value
(x: Int) => f(x)