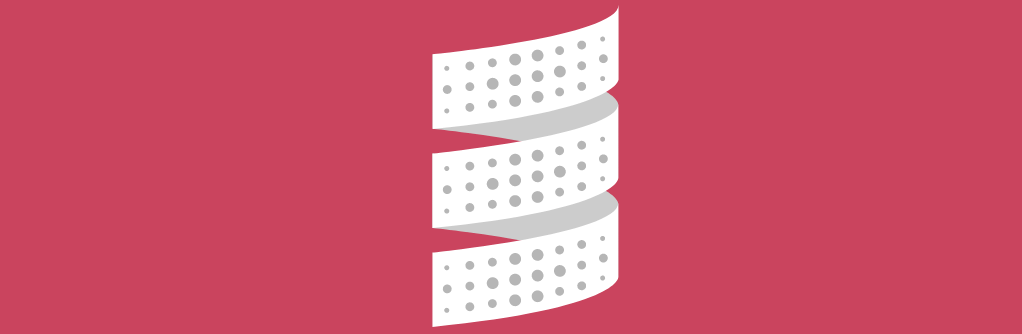
Currying is when you break down a function that takes multiple arguments into a series of functions that each take only one argument.
Example
Ex1: Write a function that takes the product of all the integers on a given interval
def product(a: Int)(b: Int): Int = { if (a > b) 1 else a * product(a + 1)(b) } product(2)(3)
Ex2: Write a function that takes the product of the factorials of all the integers on a given interval
def fact(x: Int): Int = if (x == 0) 1 else x * fact(x - 1) def productFacts(a: Int)(b: Int): Int = { if(a > b) 1 else fact(a) * productFacts(a + 1)(b) } productFacts(2)(3)
Ex3: Write a general function which generalizes both sum and product?
def fact(x: Int): Int = if (x == 0) 1 else x * fact(x - 1) def sum(x: Int, y: Int): Int = x + y def product(x: Int, y: Int): Int = x * y def mapReduce(f: Int => Int, compose: (Int, Int) => Int, zero: Int)(a: Int, b: Int): Int = { if(a > b) zero else compose(f(a), mapReduce(f, compose, zero)(a + 1, b)) } def productFacts: (Int, Int) => Int = mapReduce(fact, product, 1)(_, _) def sumFacts: (Int, Int) => Int = mapReduce(fact, sum, 0)(_, _) productFacts(2, 3) sumFacts(2, 3)