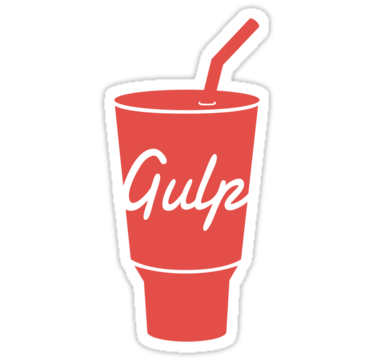
Today I will try to introduce the way to build a web client using TypeScript & AngularJs in Gulp
What is Gulp?
Gulp is a tool that bases on NodeJs (https://nodejs.org/en/). Tools like Gulp are often referred to as “build tools” because they are tools for running the tasks for building a website. The two most popular build tools out there right now are Gulp and Grunt
Overview
In this article, we will build a web client using Gulp. Web client will connect to server through Proxy. Server-side build on Scala & play framework but we don’t talk in this tutorial.
Installing Gulp
When you’ve done with installing NodeJs, you can install Gulp by using the following command on the command line:
$ sudo npm install gulp -g
The npm install command we’ve used here is a command that uses Node Package Manager (npm) to install Gulp onto your computer.
The -g flag in this command tells npm to install Gulp globally onto your computer, which allows you to use the gulp command anywhere on your system.
Mac users need the extra sudo keyword in the command because they need administrator rights to install Gulp globally.
Now that you have Gulp installed, let’s make a project that uses Gulp.
Creating a Gulp Project
Firstly create a Project folder: mkdir your_project_name
$ mkdir gulp_typescript_angularjs
Go to the project folder and start to settings now
Run npm init in project folder:
$ npm init
{ "name": "gulp_typescript_angularjs", "version": "1.0.0", "description": "Tutorial for typescript & angularjs with Gulp", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "author": "ThucNd", "license": "ISC" }
Is this ok? (yes)
You can see a new package.json file for your project which stores information about the project, like the dependencies used in the project
we can install a Gulp into the project by using the following command:
$ npm install gulp --save-dev
we’ve added –save-dev which tells the computer to add gulp as a dev dependency in
package.json
{ "name": "gulp_typescript_angularjs", "version": "1.0.0", "description": "Tutorial for typescript & angularjs with Gulp", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "author": "ThucNd", "license": "ISC", "devDependencies": { "gulp": "^3.9.0" } }
if you check the project folder, you should see that Gulp has created a node_modules. You also see a Gulp folder within node_modules
Determining folder structure
Gulp is flexible enough to work with any folder structure. You should understand how it working before tweaking it for your project.
For this article, I will use following the structure:
we will use app folder for development purposes. All source code will be placed in the app folder.
You can also install external libraries by using bower. It will be placed in the app too. we will talk it in next steps.
Now let’s begin by creating your first Gulp task
Writing Gulp Task
The first step to using Gulp is to require it in the gulpfile.
var gulp = require('gulp');
we tells Node to use Gulp module. We assign its contents to variables gulp
we can now begin to write a gulp task with gulp variable. The basic syntax of a Gulp task is:
gulp.task('task-name', function() { // Stuff here });
task-name refers to the name of tasks, which would be used whenever you want to run your Gulp task
To test it out, we can try to make a “hello-task” that says “hello world”
gulp.task('hello-task', function(){ console.log('hello world'); });
we can run this task with gulp hello-task on the command line:
$ gulp hello-task
The command line will return a log that says hello world:
[14:00:11] Using gulpfile ~/typescript/gulp_typescript_angularjs/gulpfile.js [14:00:11] Starting 'hello-task'... hello world [14:00:11] Finished 'hello-task' after 105 μs
Scripts task
Before configuring Scripts task, we need to install modules by running command line
$ npm install gulp-uglify gulp-rename --save-dev
gulp-uglify – minify files
gulp-rename – rename file
you can see 2 new dependencies in package.json and these modules in a node_modules folder
We assign its contents to variables
uglify = require('gulp-uglify')
rename = require('gulp-rename')
Add a new Scripts task in gulpfile.js
gulp.task('scripts', function () { // Source js gulp.src(['app/scripts/**/*.js', '!app/scripts/**/*.min.js']) // rename all file to .min .pipe(rename({suffix: '.min'})) // compile all file .pipe(uglify()) .pipe(gulp.dest('app/scripts')); });
gulp.src – Source path of javascript. /**/*.js – all files have type .js in any folders in app/scripts folder. we can use ! to ignore all files with type .min.js
pipe(rename({suffix: ‘.min’})) – call rename function to change all file name to filename.min.js
pipe(uglify()) – call uglify() function to minify all files
gulp.dest – Destination path of javascript.
You can see results by running command line
$ gulp scripts
In scripts folder, we can see new .min.js files which minified
Build task
Above task, we’re talking about the way to minify javascript files. But we won’t use javascript directly. In the tutorial, we write typescript instead of javascript as flow below
typescript -> angularjs -> minify
we need to install 2 more modules by running command line
$ npm install gulp-typescript-angular gulp-typescript --save-dev
gulp-typescript – A typescript compiler
gulp-typescript-angular – class-based development in AngularJS with TypeScrip
We can assign its in gulpfile.js
typescriptAngular = require('gulp-typescript-angular') typescript = require('gulp-typescript')
Add new a build task
gulp.task('build', function () { return gulp.src('app/scripts/**/*.ts') .pipe(typescript()) .pipe(typescriptAngular({ decoratorModuleName: 'web' })) .pipe(gulp.dest('app/scripts')); });
pipe(typescript()) – call typescript() function to compile
pipe(typescriptAngular()) – call typescriptAnguar() function to convert typescript to anguarjs
you can see results by running command line
$ gulp build
In scripts folder, you can see both .ts files and angularjs files
HTML task
Add new a HTML task to tell Node about HTML source
gulp.task('html', function () { gulp.src('app/**/*.html'); });
Run
$ gulp html
Default task
To make things consistent, we can also build the same sequence with the first group. Let’s use default as the task name this time:
gulp.task('default', ['scripts', 'html', 'build']);
Run
$ gulp
You can see Scripts, HTML and build task will run into Gulp command. We don’t need to run command for each time
Watch task
Watching these changes. This is very useful. At the moment, we’re needing to run command line for each time of tasks. The good news is that Gulp can watch for changes and automatically run the tasks.
Add a watch task:
gulp.task('watch', function () { gulp.watch('app/scripts/**/*.js', ['scripts']); gulp.watch('app/scripts/**/*.ts', ['build']); gulp.watch('app/**/*.html', ['html']); });
Update Default task
gulp.task('default', ['scripts', 'html', 'build', 'watch']);
This time when you run gulp it will keep running and watch for changes in the scripts/j, HTML folders and run the scripts and HTML tasks when a change is detected in their respective folder.
Browser-sync Tasks
Browser Sync helps make web development easier by spinning up a web server that helps us do live-reloading easily
we’ll first have to install Browser sync
$ npm install browser-sync http-proxy-middleware --save-dev
browser-sync – Live CSS Reload & Browser Syncing
http-proxy-middleware – proxy middleware for connecting, express and browser-sync
Assign it’s in gulpfile.js
browserSync = require('browser-sync') reload = browserSync.reload
Add a new browser-sync
gulp.task('browser-sync', function () { // Connect to Proxy server var context = ['/api']; var options = { target: '
', changeOrigin: true, ws: true, pathRewrite: { '^/api' : '/' } }; var proxy = proxyMiddleware(context, options); browserSync({ server: { baseDir: "./app/", middleware: [proxy] } }); });
we’ll connect to server side through Proxy.
context = [‘api’] URL with “/api”
target: Address of proxy server
BaseDir: Our source path. You should create an index.html file as main running
pathRewrite: replace ‘/api’ to ‘/’. When client call Ajax with Url: ‘api/accounts/’ then it’ll connect to server and call URL: ‘http://localhost:9000/accounts’
Update default task
gulp.task('default', ['scripts', 'html', 'browser-sync', 'build', 'watch']);
Run
$ gulp
Gulp run and automatic open your browser and it’ll access to an index.html file which put in the app folder